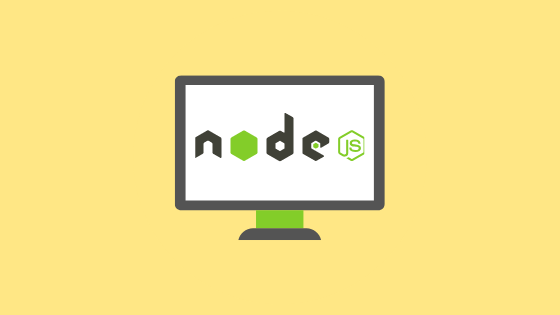
Getting Started with Node
What is JavaScript?
There's a burning question you might be having at this point ā and it might be something like
Well, what is Node?
And to answer that question, we have to understand what JavaScript is.
JavaScript is a programming language that was designed with the purpose of enabling web pages (think HTML) to engage with a user and to interact with their actions.
It meant that you could do things like
- Responding to a user clicking on a button
- You could time actions to occur after a specified amount of time
- You could retrieve and validate their entries on input fields
- You could do a whole slew of things that focused upon the user
When you look at these operations JavaScript could perform, it becomes clear that JavaScript was made with a specific goal in mind ā which is to interact with a user on a web page.
We could go in-depth with JavaScript and how it works ā but we'll save that topic for a future post.
What is Node?
Given what we know about JavaScript from the section above, we're all set to understand where Node comes into play.
Borrowing from what the NodeJS website has to say
As an asynchronous event-driven JavaScript runtime, Node.js is designed to build scalable network applications
Now what can we learn from this description?
First, it tells us that Node is a runtime for JavaScript.
What that means is that when you're running a program using Node, you're just executing JavaScript within the environment that Node provides to you.
You can think of the Node runtime environment as being a substitute for what would typically be the browser environment.
Remember, JavaScript was originally intended to run within a browser ā it wasn't meant nor geared towards being run by a non-browser environment.
Second, Node was designed to enable you create scalable network applications.
In essence, this means Node enables you to write servers using JavaScript (i.e. servers are network-based applications).
Let's recall, JavaScript was intended for client-side interaction. It wasn't intended for creating servers nor capabilities that interact with an operating system.
You can see that the existence of Node turns that whole paradigm regarding JavaScript on its head.
Rather than being confined to its original purpose of handling interactions on a website, JavaScript can now be used to serve and deliver those web pages ā among a whole other slew of server-side capabilities.
Installing Node
Now that we know about Node and JavaScript, let's move onto installing Node and using it.
If you're on Windows
You can head over to the NodeJS homepage to download the latest LTS version (Long Term Support). All this means is this version of Node will be maintained much longer than the more frequent builds.
If you're on MacOS or Linux
We can use a neat little tool called nvm
ā also known as Node Version Manager.
It's a command-line tool that helps you quickly install and manage different versions of Node on your computer.
You can also download Node from the NodeJS homepage if you prefer to do that instead.
To install nvm
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.35.3/install.sh | bash
Verify that it installed using
nvm --version
And then install the latest version using
nvm install node
Checking that Node is installed
You can check which version of Node you installed using
node --version
Creating a Node Application
Let's see, we've done the following
āļø Understood what JavaScript is
āļø Understood what Node is
āļø Learned that Node is able to execute JavaScript code
āļø Installed Node on our computer
We're all set to create a small Node application. Our app will prompt the user for some information and display it back to them.
I'm going to write my code in TypeScript. The same code will still function in JavaScript ā though you may need to make some changes to it.
We'll need the prompts
package for our application to be able to ask a user to enter data. Run the below to install it.
npm install prompts
Adding the Code for our Node Application
Let's create a file called app.ts
and get to coding.
In the following snippet, we're importing the prompts
package for use. We're also declaring our mainProgram()
function and then we're calling it at the bottom of the file.
import prompts from 'prompts';
/** Our main program */
const mainProgram = async () => {
// Declare our questions
const questions = [
{
type: 'text',
name: 'name',
message: 'What is your name?'
},
{
type: 'text',
name: 'email',
message: 'What is your email?'
},
{
type: 'number',
name: 'age',
message: 'How old are you?'
},
{
type: 'text',
name: 'favoriteShow',
message: 'What is your favorite TV show?'
},
];
// Get the responses for our questions
const responses = await prompts(questions);
// Assign the answers to variables
const { name, email, age, favoriteShow } = responses;
};
// Start our main program
mainProgram();
If your code is in JavaScript, you can execute it using
node app.js
If your code is in TypeScript, you'll need to compile before doing the above (Hint: use tsc app.js
).
Display Feedback to the User
At this stage, our app asks the user for responses to some question ā great. But we don't do much with it yet.
Let's fix that by adding a function that constructs a response using their answers.
Add the following code at the end of your mainProgram()
function.
...
// Assign the answers to variables
const { name, email, age, favoriteShow } = responses;
// Call our information handler
handleEnteredInformation(name, email, age, favoriteShow);
And add a new function below the mainProgram()
function
/** Handles the passed information */
const handleEnteredInformation = (name, email, age, favoriteShow) => {
// Create our text response
const response = `
Hi ${name}!
Thanks for telling us all about yourself.
We now know your email is ${email}.
You're currently ${age} years old and your favorite show is ${favoriteShow}.
It was a pleasure hearing from you.
`;
// Display the response to the user
console.log(response);
}
And voila!
Run your application again (you'll need to recompile it if you're using TypeScript) and you'll see the responses you entered read back to you in a neat little paragraph.
You've done it ā you learned about JavaScript and Node and used that information to create a small Node application.
The possibilities from here are endless.