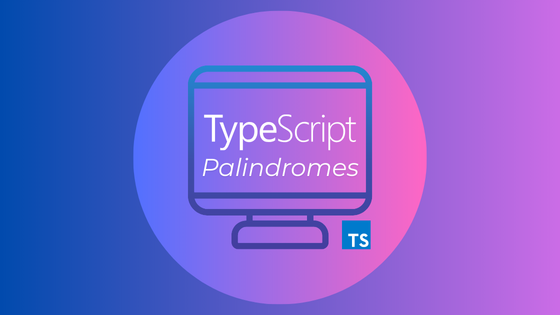
Is it a Palindrome? Or is it not?
What's a Palindrome?
You might be asking yourself...what is a Palindrome? And why do I need to write some code that will check if a string is one?
To start, a Palindrome is a word that is spelt the same backwards as it is forwards. Some examples are
- Racecar
- Level
- Civic
Each of these words result in the same word, even if you reverse their order; the resulting word is still the same as it had been prior.
It's a typical LeetCode-esque question meant to help uncover how you address an unfamiliar technical challenge and reason about it.
For this post, we'll implement a quick, and relatively easy to understand, algorithm to help us check if a provided string is indeed a Palindrome.
Our `isPalindrome` Function
Now that we know what a Palindrome is, we can get to defining our function. We will call it isPalindrome
since it makes sense.
Its input argument will be a single string that we run our algorithm against to determine if it's a Palindrome. Our return value will just be a simple boolean
on whether or not the string was indeed a Palindrome.
We also have a base-case that we can define at this time. For all intents and purposes, a single-character input string is technically a Palindrome, since it is the same forward and backward. So we'll include that as well.
The function signature will look like this
function isPalindrome(input: string): boolean {
// A single-character string is technically a Palindrome
if (input.length === 1) {
return true;
}
}
Let's move onto the next section for a breakdown of our approach, and implementing that in the code.
The Approach to Identifying a Palindrome
To figure out how we can go about checking for a Palindrome, let's call back to what a Palindrome is, and the implications that has.
A Palindrome is the same forwards as it is backwards. Great! And what we can glean from that, is that a Palindrome is a mirror-image of a particular set of characters.
From that, we can come to the realization that the letter at the beginning of the Palindrome should have a matching counterpart at the end of the Palindrome. If we were to remove those two characters, then the subsequent leftmost and rightmost characters should be equivalent as well.
If a word doesn't satisfy this comparison; this existence of a counterpart equivalent character at the same distance from the opposite side of the word — then the word can't possibly be a Palindrome.
Considering the above, this is the approach we'll take in the code
- 1 . Convert our input to an array of its characters using
input.split('')
. We'll call the variable for itinputSplit
- 2 . Using a
while
loop, where the exit condition will be whether or notinputSplit
still has a non-zero length
- 3 . Within the loop, we'll get the leftmost and rightmost characters from
inputSplit
. We'll compare these two characters for equivalency. If they are not equivalent, then we know our input is not a Palindrome. If they are indeed equivalent, we'll continue onto the next iteration, until we are left with 1 or 0 characters
- 4 . By the end of the loop, if we have not encountered a scenario where the leftmost and rightmost characters are not equivalent, then our input was indeed a Palindrome
So let's code that up!
// Our Palindrome-validating function that accepts a string
function isPalindrome(input: string): boolean {
// Our approach to checking this input string for the quality of being a palindrome will be the following
// 1. A single-character string is technically a Palindrome. We'll return true immediately if this is the case
// 2. We'll split the input into an array of its characters
// 3. We'll utilize a `while` loop to check the leftmost and rightmost characters of the input string - modifying it in-place as we do so using shift and pop
// There's a base-case to handle, that once we reach a length of 1, we can consider the number a palindrome
// If at any point, our leftmost and rightmost values are not equivalent, then the input number is not a palindrome
// A single-character string is technically a Palindrome
if (input.length === 1) {
return true;
}
const inputSplit = input.toString().split('');
// Our exit- condition will be once our input split has no characters left to check
while (inputSplit.length) {
// If we've reached a length of 1 after popping and validating
// the leftmost and rightmost values, then we have a palindrome
if (inputSplit.length === 1) {
return true;
}
const leftmost = inputSplit.shift();
const rightmost = inputSplit.pop();
// The leftmost and rightmost values need to be the same for it to have been a valid palindrome
if ((leftmost === rightmost) === false) {
return false;
}
}
// Down here, we handle the case of a input string like `abba`
// Where we would have validated both the leftmost and rightmost
// values, but we end up breaking out of our while-loop due to 0 characters left
// Since we didn't encounter the failure conditions, then this had to be a palindrome
return true;
}
And there we have it! The comments in the code help explain what's going on at each step, and what happens as a result of it.
See the attached GitHub Gist for the full code file.