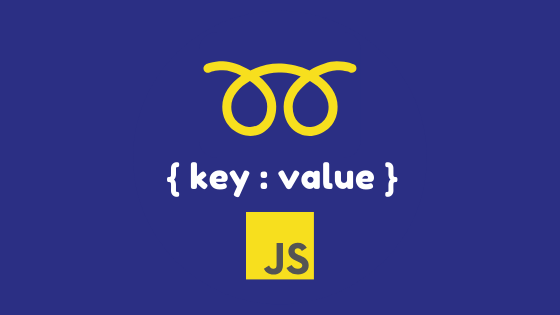
Looping Through an Object's Properties
What's an Object and Why Loop Through It?
In programming, an object is a data structure that is able to hold information.
Objects are typically used to store that data in a key-value format. This means, that in order to access a specific piece of data (let's call this a value) that the object contains, you must know the key to access it with.
These key-value pairs together are known as properties of the object.
An example is this — an object that stores information about a car
// This is an object representing a car
const randomCar = {
model: 'Model S',
manufacturer: 'Tesla',
exteriorColor: 'Black',
interiorColor: 'Tan',
numberOfWheels: 4
};
Some of the keys in this object are model
, manufacturer
, exteriorColor
, and so forth.
At times, you may want to access each property of an object but you may not necessarily know the key for each property in the object.
That's where looping through an object comes in handy and here are a few ways to do it.
The "for...in" Loop
A quick way to loop through the keys in an object — like ours above — is using the for...in
loop.
It's simple and can easily get you the keys of an object which you then use to get the value for that key.
Here's how it looks for our randomCar
object from above
// This gets each key in the object
for (let key in randomCar) {
// A caveat is we will get inherited properties as well
// So we should check this property really is only ours
if (randomCar.hasOwnProperty(key)) {
// Let's get the value for the key
const value = randomCar[key];
console.log(`The key "${key}" has a value of "${value}"`);
}
}
There's a little tidbit to add about using the for...in
loop, and it's that we need to check that the property we've accessed belongs directly to our object and wasn't inherited.
That's what is being done in the line of code
...
if (randomCar.hasOwnProperty(key))
...
This typically only comes into play if an object inherits from some other class. A more modern and concise approach is shown below.
A Modern Approach — Object.entries()
An even faster way of looping through an object's properties is using Object.entries()
.
You call the Object.entries()
method by passing in your object as an argument and it'll return a list of the key-value pairs available in the object.
In our usage with our randomCar
example, we'd call the Object.entries
like this
Object.entries(randomCar)
With Object.entries()
, we can retrieve both the key and value of each property — all with a single line of code.
Here's how we do that with our randomCar
object
// This gets you both the key and the value for each property
for (let [key, value] of Object.entries(randomCar)) {
// Object.entries get us our own properties
// So no need for an additional check
// We have the key and value
// and can display it immediately
console.log(`The key "${key}" has a value of "${value}"`);
}
And that's all there is to it. Hopefully these two snippets of code get you looping through objects no time.
You can read more about the for...in loop here and more about Object.entries here.