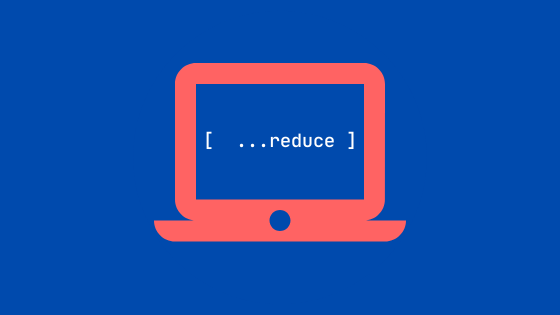
Reducing the Confusion around Reduce
What is the Reduce Method?
The reduce()
method is a function available on arrays in JavaScript.
You can use it to create complex transformations using an existing array that doesn't have to be the same size as the original array.
That part's pretty important, and one of the most useful aspects of reduce as reduce is typical used to return a single value.
That single value, however, can be any sort of data structure — be it a new array, an object, a string, etc.
Understanding the Usage of Reduce
The reduce function accepts two main arguments
- A callback function — which itself accepts a few values
- An initial value that is provided to the callback function — which can be an array, object, etc.
You can use the callback function and the initial value together to do some pretty neat stuff. The callback function accepts these arguments
- The total or accumulator as I like to think of it
- The current value of the array at that iteration
- The index of that current value
- The array that current value belongs to
The reduce function, once it completes, returns the accumulator.
Because you control what gets stored in the accumulator and what doesn't, the accumulator doesn't have to be the same size as the original array.
A List from Mozilla
Now that we know how reduce can be used on an array, let's apply it.
I'm using the list of heroes located in the members
field from Mozilla's superheroes.json.
You can copy and paste it into your file. For me it looks something like this
const heroes = [
{
"name" : "Molecule Man",
"age" : 29,
"secretIdentity" : "Dan Jukes",
"powers" : [ ... ]
},
{
"name" : "Madame Uppercut",
"age" : 39,
"secretIdentity" : "Jane Wilson",
"powers" : [ ... ]
},
{
"name" : "Eternal Flame",
"age" : 1000000,
"secretIdentity" : "Unknown",
"powers" : [ ... ];
}
];
Applying Reduce
Let's say you want only heroes that aren't immortal — and you only want their name and powers at that — your code to reduce the heroes list might look like this
const notImmortalHeroes = heroes.reduce((accumulator, currentHero) => {
// We only want heroes that aren't immortal
if (currentHero.age <= 100) {
// Create our hero object
const hero = {
name: currentHero.name,
age: currentHero.age,
powers: currentHero.powers
};
// Add this hero to our accumulator
accumulator.push(hero);
}
return accumulator;
}, []);
console.log(notImmortalHeroes);
You can see in the above code, we only really do anything inside the callback function if the hero's age is less than 100.
In addition to that, we create a new object that contains only the hero's name, their age, and their power set.
Lastly, only within that if
block do we add a hero into our accumulator.
Go ahead and run your code — you should see that your newly reduced list of heroes.
[
{ name: 'Molecule Man',
age: 29,
powers:
[ 'Radiation resistance',
'Turning tiny',
'Radiation blast' ]
},
{ name: 'Madame Uppercut',
age: 39,
powers:
[ 'Million tonne punch',
'Damage resistance',
'Superhuman reflexes' ]
}
]
And there you have it — a simplified but straightforward application of reduce.