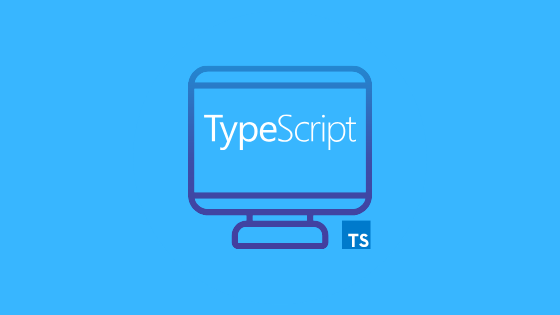
Starting a TypeScript Project
Installing TypeScript with Node Package Manager
In order to start a new TypeScript project, you'll probably want the typescript
package installed. Run the below command to do so.
npm install -g typescript
\
Once that completes, you can verify the install was successful by running the command tsc --version
. If everything went smoothly, you'll see something like the below, of course with whatever version you happened to install.
tsc --version
Version 3.8.3
A Quick Little Program
Let's make some magic happen. Create a new file — app.ts — and add the following code in the file.
const accomplishment = () => {
console.log(`You just made this program! High five!`);
}
accomplishment();
Great. We've got our TypeScript file, but it isn't something that Node can natively run. That's no problem though, we've got TypeScript installed to compile it to JavaScript — something Node does understand.
\ Run the below to compile app.ts.
tsc app.ts
\ You should see a new file — app.js — was created at that point — right next to your existing app.ts. You can now use node to natively run that with a simple...
node app.js
and you'll see the text "You just made this program! High five!" displayed in the terminal.
\ It's a small little program, literally just a few lines of code, but a program nonetheless — and a TypeScript one at that.
Creating the TypeScript Project
Navigate to the directory you want to create your new TypeScript project in and run the command
tsc --init
\
This will initialize that directory with a tsconfig.json
file. That file tells the TypeScript compiler that this directory contains a TypeScript project, and that files within the directory can be compiled from TypeScript to JavaScript.