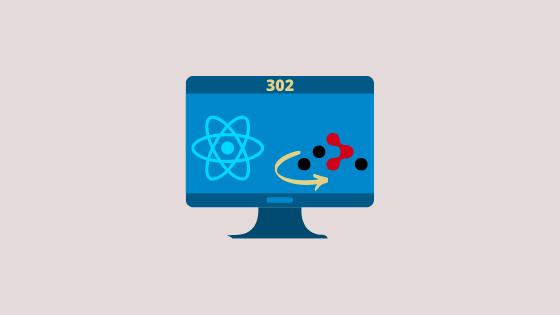
Using Redirects in React Router
What are Redirects?
When you're working on an application, you probably have quite a few routes set up.
If you're doing user authentication and setting up accounts for them, you might have
- A route for logging in
- A route for signing up
- A route for resetting your password, etc.
And there may be times where you want to redirect a user from one place to another — like to their profile once they've signed up.
This is what redirects are and frameworks implement them differently. Today we'll do so with React and React Router and it'll look like this once done.
When are Redirects used?
You might want to use a redirect following a specific action your user does or if they've landed somewhere they don't need to be.
An example of redirecting after a specific action might be the following
- A user goes to your app or site
- They go to your sign up route and complete the sign up process
- After a few seconds, you might want to redirect them elsewhere — like their account
If you're using React Router, redirects are made simple and intuitive.
Not set up with React Router yet? See this 5-minute post on baking it into your app Figuring out React Router.
Usage of Redirect
Assuming you've got React Router installed in your project and a <BrowserRouter>
setup in your project, you can use redirects almost immediately.
First import the <Redirect>
component from react-router
into your component, like so
import { Redirect } from "react-router-dom";
Now we'll use the redirect component.
The redirect component accepts a few props but we'll only be using the to
prop to tell it where we want it to take us. This is its general usage.
// A conditional determines when to redirect
if (shouldRedirect) return <Redirect to="/elsewhere" />;
This would redirect you to a route /elsewhere
in your app.
Coding Up the Redirect
For your app, set up a state variable and conditional to tell your component when the redirect should be occur in your component.
When that condition is met, your component will execute the redirect. See this example below.
const SignUp = () => {
const [shouldRedirect, setShouldRedirect] = useState(false);
const [second, setSecond] = useState(5);
// An interval that will execute every 1 second
const fiveSecondRedirect = setInterval(() => {
// Decrement our second variable
// Remember it starts at 5 seconds
setSecond(second - 1);
// Once we hit 0 seconds
// Let's redirect and clear the interval
if (second == 0) {
setShouldRedirect(true);
clearInterval(fiveSecondRedirect);
}
}, 1000);
// Redirect to the login page when `shouldRedirect` is true
if (shouldRedirect) return <Redirect to="/login" />;
// Otherwise, display our normal content
return (
<div>
<p>Here is where we'd put our Sign Up form ✍️</p>
<p> After 5 seconds, we'll redirect back to the login page</p>
<p>{second}...</p>
</div>
);
};
In the above, we're using a redirect to take us from our Sign Up component to the path /login
.
We only redirect when the variable shouldRedirect
is true, and we only update that variable to true after 5 seconds. It starts off as false
in the component.
And we've done it! That's how redirects work and you can see in the gif from above how it looks in practice.